What is Setapp?
As a Macbook user,especially as a developer, we probably need lots of productive applications and tools to work with Macbook. However, there are many applications you need to buy before using them, or you can start a free trial and buy it later. Also, there are also many excellent applications you may not know and download.
It’s really unreasonable to buy many applications which you only use them for several times. Is there any way like Netflix that I can subscribe it and use all applications.
Setapp is just like its name, which is a set of applications. By downloading in your Macbook, you can easily reach whenever you need it.
Setapp will charge you monthly subscription fee with $9.99 just like Netflix, and you will have unlimited access to all the applications it has. Of course, Ads free. Below is the screenshot that Setapp has.
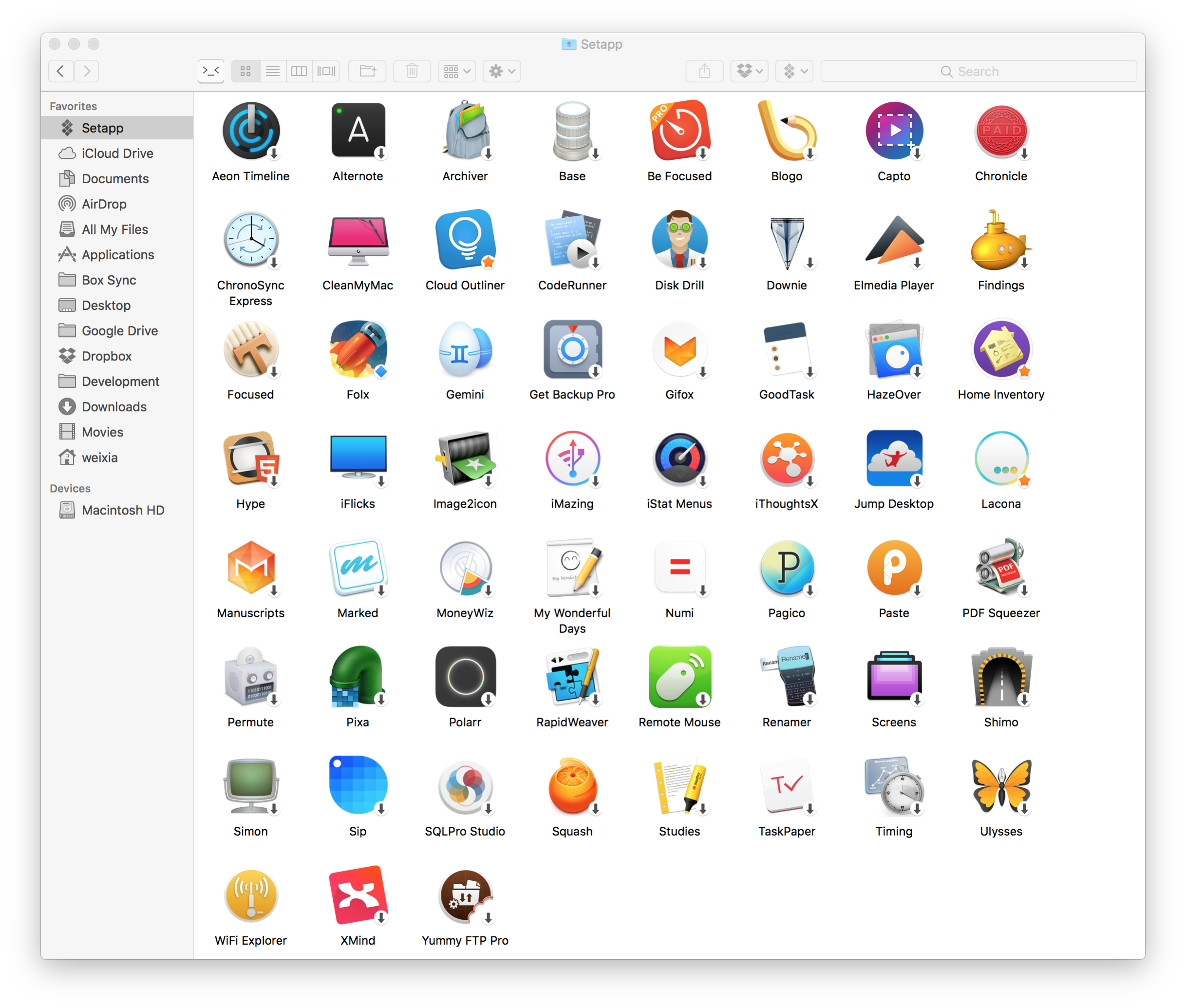
At this moment as January 10, 2017, Setapp is still a beta version, and you have free access until March 2017. So, it is a good time to try it without paying any fee.
How it works?
After installing Setapp, it has its own folder. All you need to do is to download the applications you need inside.
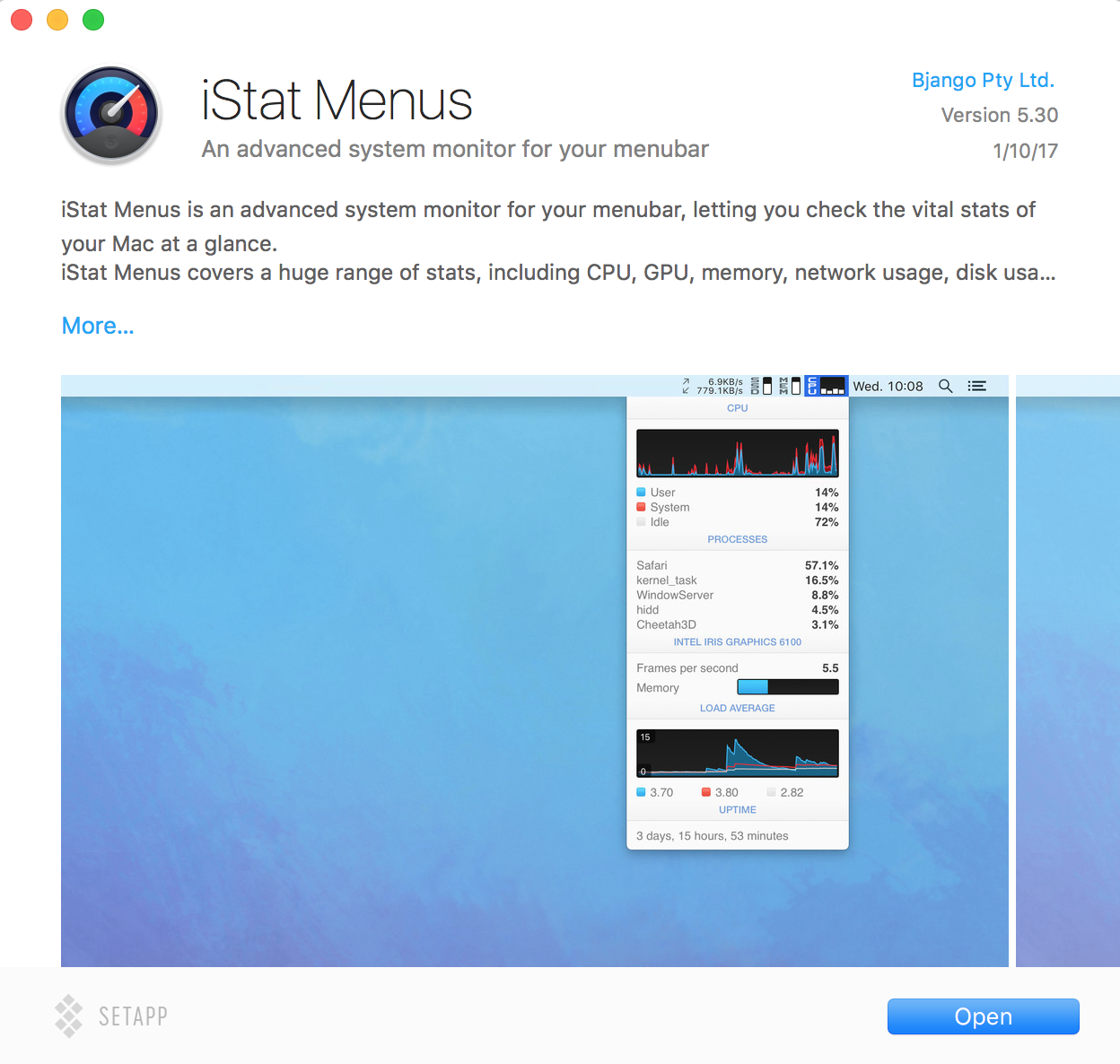
Above is the glance of iStat Menus, it has screenshots and a brief introduction. Click Open and downloaded, you can easily access to this application. The applications also support Spotlight Search like below.
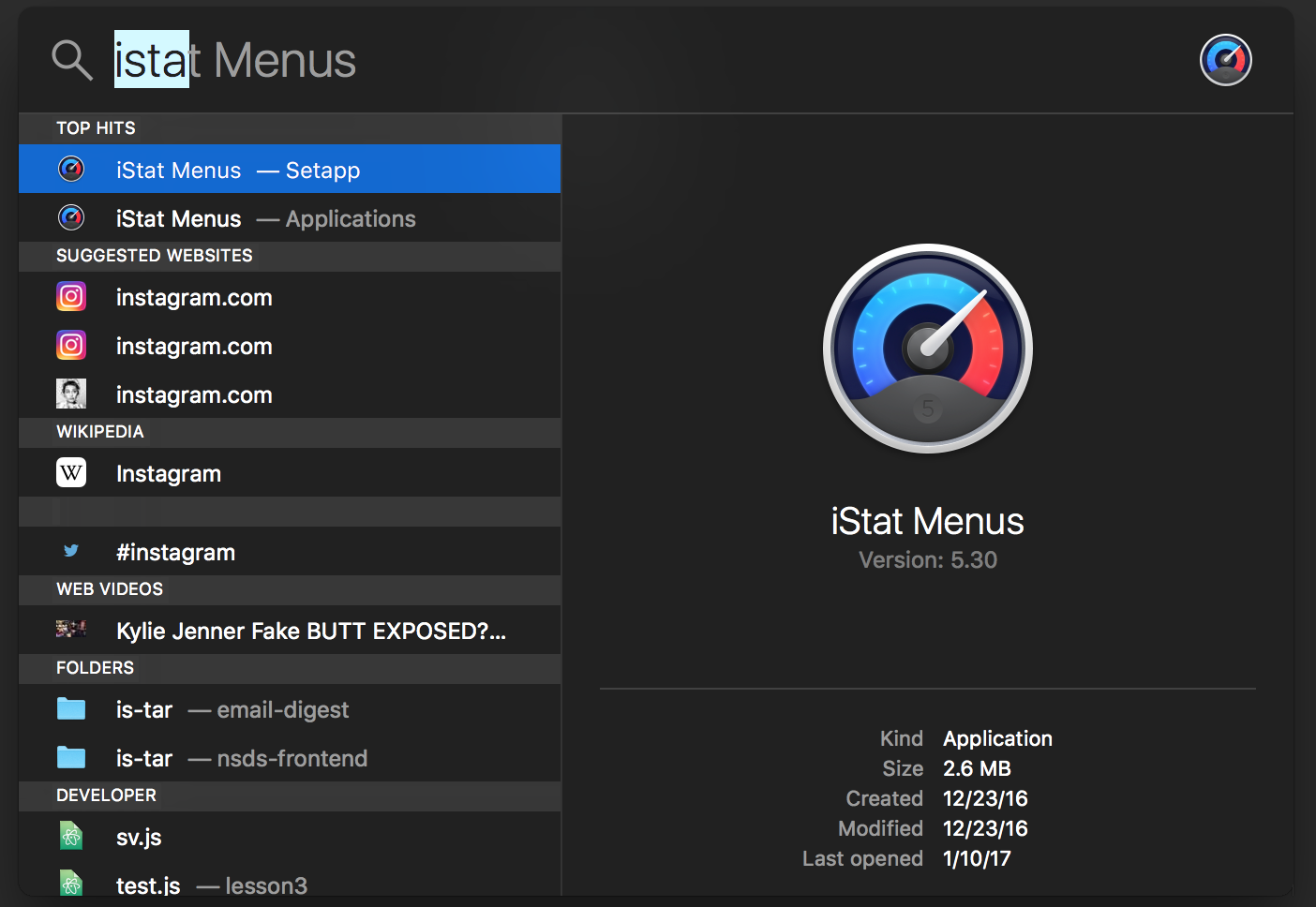
Want to give Setapp a try?
If you feel like to give Setapp a try, you can use my invitation link to download the app. All Beta users get free Setapp access until March 1st.
Please note I won’t get any benefit from you if you use my link, and you can get instant approval of using Setapp.
The invitation link is only available for first five friends.
This is the end of post